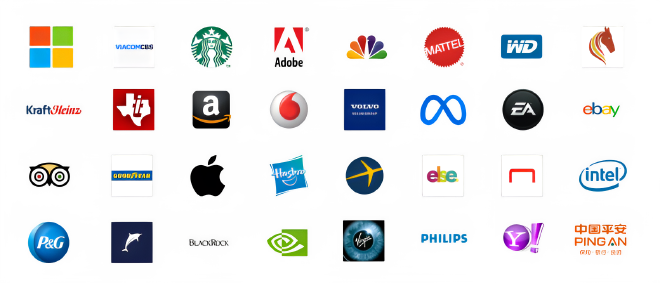
The stock market is a dynamic and complex ecosystem where shares of publicly traded companies are bought and sold. This constant exchange of ownership stakes, driven by investor expectations and company performance, has a profound influence on global economies and the financial fortunes of individuals and institutions alike. It’s a place where risk and reward are intertwined, where fortunes can be made and lost, and where the daily ebb and flow of prices reflects the collective hopes and fears of the investing world.
Understanding the Stock Market Fundamentals #
The stock market, also known as the equity market, is a crucial component of the modern financial system. It provides a platform for companies to raise capital by selling ownership shares (stocks) to investors. These investors, ranging from individual retail traders to large institutions, buy and sell stocks based on their expectations of the company’s future performance. When a company performs well, its stock price typically rises, benefiting its shareholders. Conversely, if a company underperforms, its stock price may fall.
Several key factors influence stock prices, including:
- Company Performance: Earnings reports, revenue growth, profitability, and new product launches are primary drivers.
- Economic Conditions: Interest rates, inflation, economic growth, and unemployment rates can all impact investor sentiment and market valuations.
- Industry Trends: Developments and trends within a specific industry (e.g., technology, healthcare, energy) can affect the stocks of companies in that sector.
- Investor Sentiment: Market psychology and investor confidence (or lack thereof) play a significant role, sometimes leading to irrational exuberance or panic selling.
- Geopolitical Events: Political instability, wars, trade disputes, and other global events can create market volatility.
Investing Strategies and Risk Management #
There are numerous approaches to investing in the stock market, each with its own level of risk and potential reward. Some common strategies include:
- Value Investing: Identifying undervalued companies with strong fundamentals that the market has overlooked.
- Growth Investing: Focusing on companies with high growth potential, even if they are currently expensive.
- Dividend Investing: Investing in companies that pay regular dividends to shareholders.
- Index Investing: Buying and holding a diversified portfolio of stocks that mirrors a specific market index (e.g., the S&P 500).
Successful investing requires careful risk management. Diversification (spreading investments across different asset classes and sectors), setting stop-loss orders, and having a long-term investment horizon are important strategies for mitigating risk.
Building a Stock Scanner in R #
R, with its powerful data analysis and visualization capabilities, is an excellent tool for building a custom stock scanner. A stock scanner allows you to filter and identify stocks that meet specific criteria, helping you to find potential investment opportunities quickly.
Here’s a basic outline of how to create a simple stock scanner in R using the quantmod
package (a popular package for financial analysis):
# Install and load necessary packages
if(!require(quantmod)){install.packages("quantmod")}
if(!require(dplyr)){install.packages("dplyr")}
library(quantmod)
library(dplyr)
# 1. Define a list of stock symbols
symbols <- c("AAPL", "MSFT", "GOOG", "AMZN", "TSLA")
# 2. Download historical stock data
getSymbols(symbols, from = "2023-01-01", to = Sys.Date())
# 3. Create a function to calculate technical indicators
calculate_indicators <- function(data) {
data$SMA_50 <- SMA(Cl(data), n = 50) # 50-day Simple Moving Average
data$SMA_200 <- SMA(Cl(data), n = 200) # 200-day Simple Moving Average
data$RSI <- RSI(Cl(data)) # Relative Strength Index
data$MACD <- MACD(Cl(data))[,1] # MACD signal line
return(data)
}
# 4. Apply the function to each stock's data
stock_data <- lapply(symbols, function(symbol) {
calculate_indicators(get(symbol))
})
names(stock_data) <- symbols
# 5. Filter stocks based on criteria (example)
filtered_stocks <- lapply(stock_data, function(data) {
# Get the most recent data point (last row)
last_data <- tail(data, 1)
# Filter criteria:
# - Current price is above the 50-day SMA
# - Current price is above the 200-day SMA
# - RSI is above 50 (indicating bullish momentum)
if (Cl(last_data) > last_data$SMA_50 &&
Cl(last_data) > last_data$SMA_200 &&
last_data$RSI > 50) {
return(TRUE)
} else {
return(FALSE)
}
})
# 6. Print the filtered stocks
print(symbols[unlist(filtered_stocks)])
# Example to create a dataframe (more versatile for complex filtering)
all_data <- data.frame()
for (symbol in symbols){
temp_data = calculate_indicators(get(symbol)) |> as.data.frame()
temp_data$symbol = symbol
temp_data$date = row.names(temp_data)
row.names(temp_data) <- NULL
all_data <- rbind(all_data, tail(temp_data,1))
}
#Using DPLYR
all_data |> filter(RSI > 50 & MACD > 0)